The idea is that if a player pushes the analog control in any particular direction and holds it there, his character will move in either a straight line, or a circle (assuming it doesn't hit any obstacles). So let's assume that the best way to predict the players position is to assume that the current turning speed and linear speed will stay the same over the desired prediction time.
This method of prediction fixes the naive approach of just adding the current linear velocity times the number of seconds forward. All the player has to do to fool this prediction is run in very tight circles. The whole time the player would have a very large linear velocity, but he doesn't end up going anywhere, because of the large angular speed. So this post will show you how to take the player's angular speed into account.
So let's say P(t) is the position of the player at time t, and dt is the change in time from the last update. So the estimated velocity is
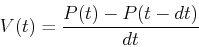
Let's call D(t) the heading direction at time t, and s(t) is the speed (scalar) at time t. Also, let's assume that the player moves mostly in the xz-plane, and the positive y-axis is the natural up vector. We will only attempt to predict the player's xz movement, since jumping, double jumping, etc. tend to make the vertical movement more erratic. So we'll calculate the speed and direction by


First, if s(t) = 0, then the predicted position is just the current position, P(t), and we're done. Otherwise the players current heading direction is

So now we can get a turning rate (angular velocity about the y-axis) as

Given that D(t) is a unit vector with no y-component, we can calculate a "left" vector with

So now that we have an angular velocity, and a linear velocity, we can extrapolate the player's position u seconds forward. If a(t) = 0, then

and

Otherwise (for non-zero a(t)), we have

Integrating this gives us

And solving for C

And so, the player's position at time t+u is given by

Once you have this set up, you'll probably notice that the predicted position jumps around quite a bit. To help alleviate this, just keep track of the current linear and angular speeds, and smooth them out over a few updates.
You can also use some fraction of their current linear and/or angular speed when evaluating a particular prediction in order to "lead" the player less (or not at all). Or you can use multipliers greater than 1 to overshoot the player.
Thanks for this. It will come in a lot of use and will save me a bunch of time. It's a better formula then one I would have written on my own.
ReplyDeleteThanks again!